单例模式实现
懒汉式
第一次调用时才实例化对象
线程不安全
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
|
public class Singleton_01 {
private static Singleton_01 instance;
private Singleton_01() { }
public static Singleton_01 getInstance(){ if (null != instance) return instance; return new Singleton_01(); } }
|
线程安全
1 2 3 4 5 6 7 8 9 10 11 12 13
| public class Singleton_02 {
private static Singleton_02 instance;
private Singleton_02() { }
public static synchronized Singleton_02 getInstance(){ if (null != instance) return instance; return new Singleton_02(); }
}
|
双检索
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| public class Singleton_05 {
private static volatile Singleton_05 instance;
private Singleton_05() { }
public static Singleton_05 getInstance(){ if(null != instance) return instance; synchronized (Singleton_05.class){ if (null == instance){ instance = new Singleton_05(); } } return instance; }
}
|
内部类
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| public class Singleton_04 {
private static class SingletonHolder { private static Singleton_04 instance = new Singleton_04(); }
private Singleton_04() { }
public static Singleton_04 getInstance() { return SingletonHolder.instance; }
}
|
饿汉式
1 2 3 4 5 6 7 8 9 10 11 12
| public class Singleton_03 {
private static Singleton_03 instance = new Singleton_03();
private Singleton_03() { }
public static Singleton_03 getInstance() { return instance; }
}
|
枚举
通过枚举实现单例,序列化和反序列化后持有对象哈希码相同,还是同一个对象。
1 2 3 4 5 6 7 8 9 10 11 12 13
| public enum Singleton_07 {
INSTANCE; private User instance;
Singleton_07() { this.instance = new User(); }
public User getInstance() { return instance; } }
|
校验代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| @Test public void test() throws IOException, ClassNotFoundException { System.out.println(Singleton_07.INSTANCE.getInstance().hashCode()); ObjectOutputStream oos=new ObjectOutputStream(new FileOutputStream("D:\\object.txt")); oos.writeObject(Singleton_07.INSTANCE); oos.flush(); oos.close();
ObjectInputStream ois=new ObjectInputStream(new FileInputStream("D:\\object.txt")); Object o = ois.readObject(); Singleton_07 s= (Singleton_07) o; System.out.println(s.getInstance().hashCode()); ois.close(); }
|
输出结果:
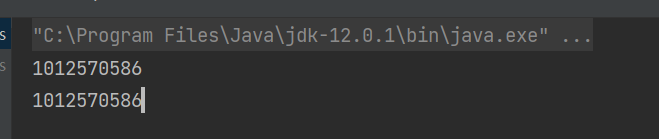